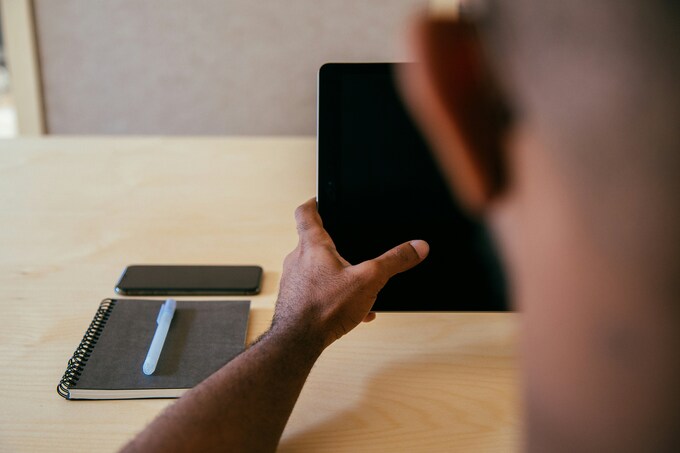
Laravel is one of the most popular frameworks for building web applications, and for good reason. It simplifies many aspects of development while providing robust security features. One powerful tool in Laravel’s arsenal is the concept of policies, which streamline authorization management.
When it comes to controlling user access to specific resources or actions within your application, having a clear and organized approach is essential. That’s where Laravel Policies come into play. They offer a clean way to manage permissions based on user roles and other criteria.
This article will dive deep into leveraging Laravel Policies for quick and efficient authorization handling. Whether you’re building a new app or enhancing an existing one, mastering this feature can elevate your project’s security and maintainability significantly. Let’s explore how you can integrate these policies seamlessly into your application architecture!
How to Create and Register Policies in Laravel
Creating and registering policies in Laravel is a straightforward process. Start by generating a policy using the Artisan command line tool. Run `php artisan make:policy PolicyName` to create your new policy class.
This command will generate a file in the `app/Policies` directory. Open this file and define your authorization logic within the methods provided, such as `view`, `create`, or `update`. Each method should return true or false based on whether the user has permission for that action.
Next, you need to register your policy. Head to the `AuthServiceProvider.php` located in the `app/Providers` folder. In this file, add an entry under the `$policies` property where you map your model to its corresponding policy class.
With these steps complete, your policy is now ready for use throughout your application’s authorization processes.
Using Gate Facade for Authorization
Laravel’s Gate facade provides a powerful way to handle authorization. It’s an elegant solution that simplifies the process of determining user permissions.
With Gates, you can define simple closures in your application. This allows for quick checks without the need to create full policies for every scenario. Simply register your gates in the `AuthServiceProvider`.
Once registered, invoking a gate is straightforward. Use `Gate::allows()` to check if a user has permission for specific actions. This returns a boolean value, making it easy to implement conditional logic throughout your application.
You can also pass parameters to gates for more complex scenarios. This flexibility means you can tailor permissions based on various criteria while keeping your code clean and readable.
Using the Gate facade not only enhances security but also boosts maintainability by centralizing authorization logic in one place.
Implementing Policies in Views and Controllers
Implementing policies in your views and controllers enhances security while maintaining a clean codebase. Start by injecting the authorization logic into your controller methods. Use the `authorize` method to check permissions before executing any critical actions.
For example, if you’re managing posts, simply call `authorize(‘update’, $post)`. This checks if the current user can update that specific post based on predefined policy rules.
In Blade templates, utilize directives like `@can` or `@cannot`. These make it easy to conditionally show content based on user permissions. For instance, wrapping an edit link with `@can(‘update’, $post)` ensures that only authorized users see it.
This approach keeps your application intuitive and responsive to different user roles. By centralizing permission checks within policies, you maintain organization and clarity throughout your code.
Advanced Techniques for Authorization with Policies
Laravel provides a robust framework for fine-tuning authorization through advanced policy techniques. One powerful approach is using dynamic policies, which allow you to define rules based on user attributes or specific resource states.
Another technique involves using closures within your policy methods. This flexibility lets you write custom logic without cluttering your codebase with repetitive checks across different controllers.
Consider leveraging a combination of multiple policies for sophisticated scenarios. By grouping related actions under a single policy class, you maintain organization and clarity in your authorization layer.
You can also implement “before” callbacks in your policies to handle common checks before any other method runs. This helps streamline the process by reducing redundancy and centralizing permission handling.
Utilize Laravel’s built-in features like route model binding alongside policies to enforce access control seamlessly throughout your application.
Conclusion
Laravel policies offer a powerful way to manage authorization in your applications. By defining clear rules for user actions, you can enhance security and maintain control over sensitive data.
Creating and registering policies is straightforward, allowing you to set up specific permissions with ease. The use of the Gate facade simplifies the authorization process further, making it accessible throughout your application.
Integrating these policies into views and controllers ensures that your logic remains clean and organized. You can easily apply restrictions where necessary without cluttering your controllers or views with complex conditions.
For those looking to take their authorization strategies further, advanced techniques like policy methods for custom actions can add more flexibility to your codebase.
By embracing Laravel policies effectively, you’ll be on a path towards building robust applications that prioritize user roles and permissions seamlessly. This approach not only enhances security but also improves the overall experience for end-users by tailoring access based on their needs.