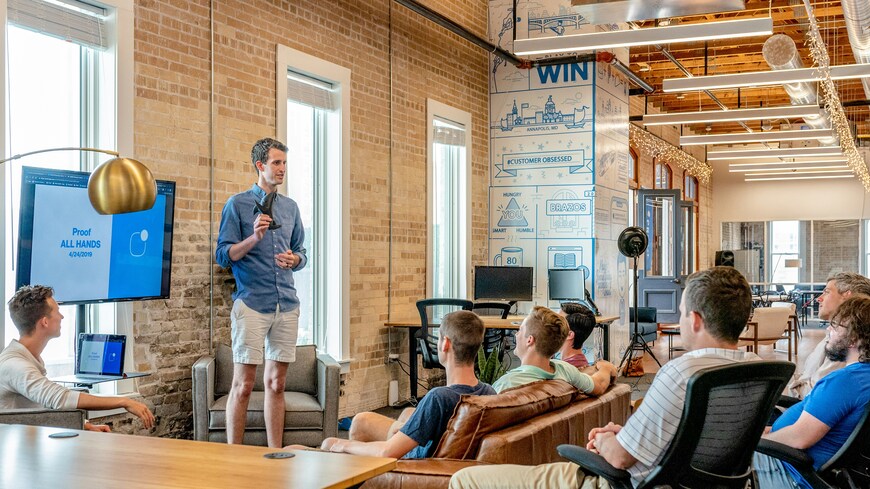
Laravel is a powerful PHP framework that has gained immense popularity among developers for its elegant syntax and robust features. As your application grows, however, you might notice performance issues creeping in—especially when it comes to database queries. This is where the magic of database indexing comes into play.
Imagine having a library filled with countless books. Without an organized index, finding a specific title would be a daunting task. Similarly, when your Laravel application interacts with large databases, not utilizing proper indexing can lead to slow response times and frustrated users.
Understanding database indexing strategies can drastically improve speed and performance in Laravel applications. Whether you’re building a small project or working on an enterprise-level system, knowing how to optimize data retrieval is crucial for user satisfaction and overall success.
Let’s dive deeper into the world of database indexing!
The Importance of Database Indexing for Speed and Performance
Database indexing is a crucial aspect of optimizing speed and performance in Laravel applications. When data grows, retrieving information without an index can become sluggish. Indexes act like a roadmap, allowing the database to locate records more efficiently.
With proper indexing, queries run faster because the database engine doesn’t need to scan every row for matches. This efficiency translates into quicker response times for users, enhancing their overall experience.
Effective use of indexes can dramatically reduce server load during peak usage times. By minimizing resource consumption, your application remains responsive even when facing high traffic.
It’s essential to strike a balance with indexing strategies. While they improve read operations significantly, improper or excessive indexing may slow down write operations due to maintenance overheads. Understanding this dynamic is key for developers aiming to enhance application performance effectively.
Different Types of Database Indexing Strategies
Database indexing can significantly enhance query performance. Various strategies cater to different needs.
B-tree indexes are the most common type. They maintain a balanced tree, allowing for quick searches, inserts, and deletions. This strategy excels in range queries.
Hash indexes use a hash table structure. They offer rapid lookups but fall short on range queries or sorting operations. Their strength lies in equality comparisons.
Full-text indexes shine when searching large text fields. They’re ideal for applications that require keyword searches rather than exact matches, enabling efficient retrieval of relevant data.
Spatial indexes cater to geographic data types, optimizing spatial queries and enhancing performance in location-based services.
Composite indexes combine multiple columns into one index. This approach speeds up complex queries involving several criteria but may increase overhead during writes due to additional storage requirements. Each strategy serves its purpose; understanding them is key to effective database optimization.
How to Implement Database Indexing in Laravel
Implementing database indexing in Laravel is straightforward. Start by defining your indexes in the migration files. Use the `index()` method when creating or modifying tables.
For example, you might write:
“`php
$table->string(’email’)->index();
“`
This command creates an index for the email column, enhancing search speed.
If you’re looking to create a composite index on multiple columns, use `unique` or `primary`. You can chain these methods together for clarity:
“`php
$table->unique([‘user_id’, ‘post_id’]);
“`
After updating your migrations, run them using Artisan commands. This applies your new indexes seamlessly to the database.
You can utilize Eloquent’s query builder with indexed columns. This ensures that queries are optimized from the start. Always monitor performance before and after making these changes to gauge their effectiveness accurately.
Common Mistakes to Avoid when Using Database Indexing in Laravel
When optimizing database performance in Laravel, it’s easy to make mistakes with indexing. One common error is over-indexing. Adding too many indexes can slow down write operations and increase storage requirements.
Another pitfall involves neglecting query profiling. Failing to analyze which queries actually benefit from indexing leads to wasted effort on unnecessary indexes.
Improperly choosing index types is also an issue. Not every scenario calls for a unique or full-text index; understanding the specific use case is crucial for effective optimization.
Forgetting about maintenance can be detrimental. Indexes require regular updates and monitoring to maintain peak efficiency, especially as data grows and changes over time. Keeping these factors in mind will help ensure your indexing strategy truly enhances performance.
Conclusion: The Impact of Database Indexing on Laravel Development
Database indexing is a crucial aspect of optimizing Laravel applications. By implementing effective indexing strategies, developers can significantly enhance the speed and performance of their applications. This enhancement leads to quicker data retrieval, which directly improves user experience.
Understanding the various types of database indexes allows for better decision-making when structuring your Laravel projects. Whether it’s single-column or composite indexes, knowing when and how to use them can make all the difference.
Implementing these strategies in Laravel is straightforward but requires attention to detail. Missteps in this process can lead to unintended slowdowns instead of improvements. It’s essential to avoid common pitfalls that may arise during implementation.
The benefits derived from proper database indexing extend beyond just faster queries; they also contribute positively to overall application efficiency. A well-indexed database reduces server load and optimizes resource usage.
Adopting robust database indexing practices will pave the way for more scalable and performant Laravel applications, making it an essential skill for any developer working within this framework.