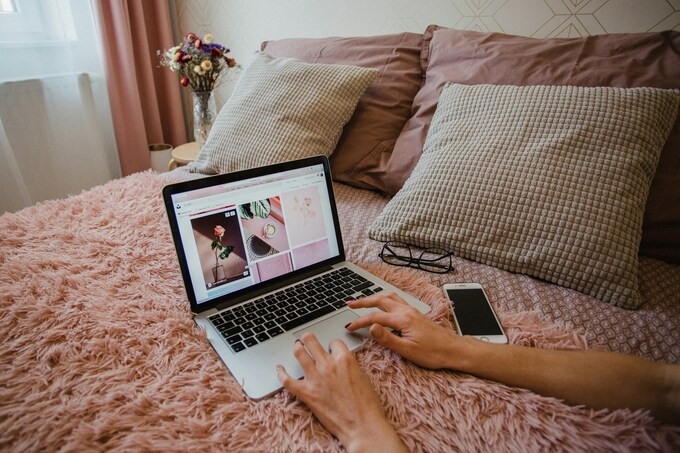
Welcome to the world of web development where creating secure forms is not just a choice, but a necessity. In the realm of Laravel, building robust and secure forms is key to safeguarding your application from malicious attacks. So, buckle up as we delve into the realm of Laravel Forms and explore best practices for fortifying them against security threats!
Common Security Threats for Web Forms
Web forms are a crucial element of any website, allowing users to input data and interact with the site. However, they also pose security risks if not properly secured. One common threat is SQL injection, where malicious code is injected into form fields to manipulate or access the database.
Another threat is cross-site scripting (XSS), where attackers inject scripts into web pages viewed by other users. This can lead to stolen data or session hijacking. Form spoofing is another risk, where attackers mimic legitimate forms to trick users into providing sensitive information.
Brute force attacks are also a concern, where automated tools repeatedly submit various inputs to guess passwords or gain unauthorized access. It’s essential for developers to implement proper validation and sanitization techniques in Laravel forms to mitigate these threats effectively.
Best Practices for Building Secure Laravel Forms
When it comes to building secure Laravel forms, there are several best practices that developers should follow. One key practice is to always validate user input on the server-side to prevent malicious data from being submitted. This can be easily achieved using Laravel’s built-in validation features.
Another important practice is to sanitize user input before storing or displaying it on the website. This helps prevent cross-site scripting (XSS) attacks by removing potentially harmful code from the input.
Implementing proper authentication and authorization mechanisms in your Laravel application can help protect sensitive form data from unauthorized access. Utilizing Laravel’s authentication system makes it easy to control access to specific routes and actions within your application.
Regularly updating your Laravel framework and dependencies is crucial for maintaining a secure environment. Updates often include security patches that address vulnerabilities discovered over time, so staying up-to-date is essential for keeping your forms secure.
Utilizing Laravel Form Requests for Validation and Sanitization
When it comes to building secure Laravel forms, one essential aspect to consider is utilizing Laravel Form Requests for validation and sanitization. This feature allows you to centralize your form validation logic within dedicated form request classes.
By creating custom form request classes, you can define specific validation rules for each input field in your form. This not only helps ensure that the data submitted meets the required criteria but also keeps your controller methods clean and focused on handling business logic.
Laravel’s form requests enable you to sanitize incoming data before it reaches your application’s core functionalities. By sanitizing user input, you can prevent common security vulnerabilities such as SQL injection attacks or cross-site scripting (XSS) exploits.
Leveraging Laravel Form Requests for validation and sanitization is a powerful way to enhance the security of your web forms and protect your application from potential threats.
Implementing CSRF Protection in Laravel Forms
Implementing CSRF protection in Laravel forms is crucial for safeguarding against cross-site request forgery attacks. By generating unique tokens for each form submission, Laravel ensures that the request originated from the legitimate user and not an unauthorized source.
To implement CSRF protection in Laravel forms, simply include `@csrf` directive within your form tags. This automatically generates a hidden input field with a token value that gets validated during form submissions.
Laravel’s built-in middleware verifies the token on each POST request, rejecting any requests without a valid token. This adds an extra layer of security to prevent malicious actions like unauthorized account changes or data manipulation.
By utilizing Laravel’s CSRF protection features, developers can enhance the overall security of their web applications and protect users’ sensitive information from potential threats.
Conclusion
Building secure Laravel forms is crucial for protecting your web application from potential security threats. By following best practices such as utilizing Laravel Form Requests for validation and sanitization, implementing CSRF protection, and staying informed about common security threats, you can ensure that your forms are robust and safeguarded against malicious attacks.
Remember that the security of your web forms is a continuous process that requires vigilance and proactive measures to stay one step ahead of cyber threats. By prioritizing security in your development process, you can build trust with users and protect sensitive data effectively.