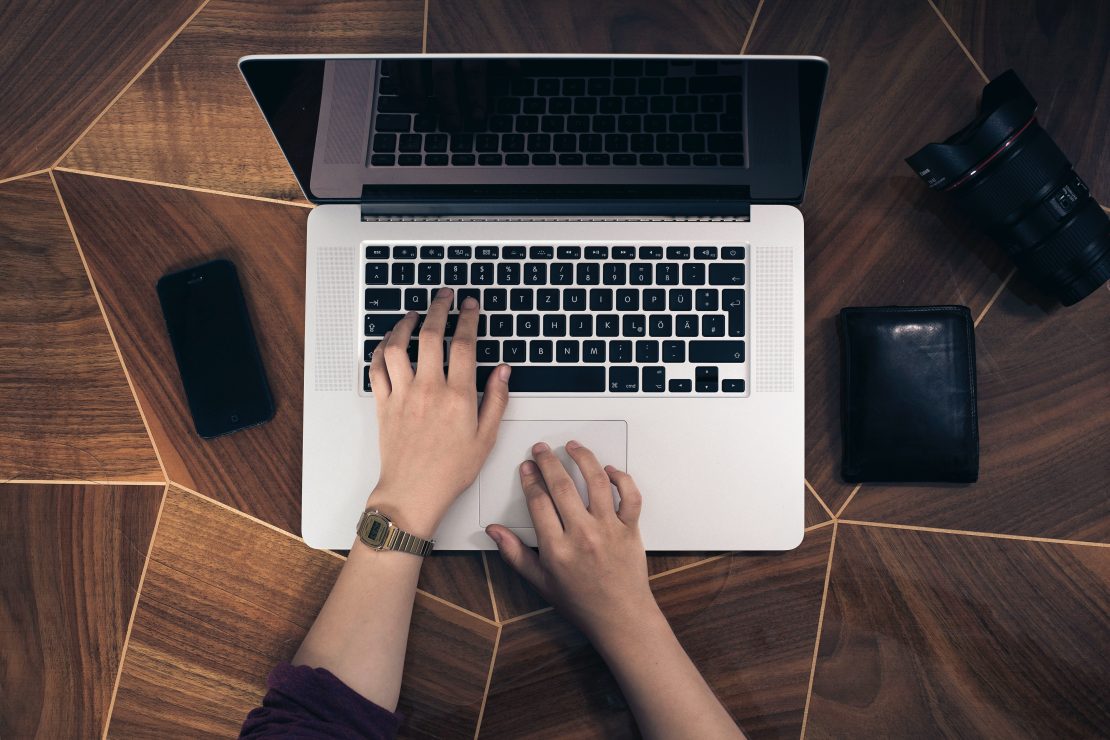
Welcome to the world of Laravel, where building scalable web applications is a breeze! But hold on tight, because along with its power and flexibility comes the challenge of handling errors efficiently. Errors are inevitable in any application development process, but with the right strategies and practices in place, you can ensure that your Laravel app remains robust and error-free.
In this blog post, we will explore the art of efficient error handling in scalable Laravel apps. We’ll delve into common types of errors you may encounter during development, discuss why effective error handling is crucial for the success of your app, and provide you with practical tips and best practices to prevent those pesky bugs from wreaking havoc.
Common Types of Errors in Laravel Apps
When working with Laravel, it’s essential to be aware of the common types of errors that can occur in your application. Understanding these errors will help you identify and resolve issues efficiently, ensuring a smooth user experience.
One common type of error is the 404 error, which occurs when a requested resource cannot be found. This can happen if a URL is incorrect or if a page has been moved or deleted. By handling this error gracefully and providing helpful feedback to users, you can prevent frustration and confusion.
Another common error is the 500 internal server error, which indicates an issue on the server side that prevents the request from being processed. This could be due to an unexpected exception or an incorrectly configured server environment. Properly logging these errors and notifying administrators will allow for swift resolution.
Validation errors are also quite prevalent in Laravel apps. These occur when user input fails validation rules defined in your application. Handling these errors by displaying clear messages to users will improve their experience and guide them towards correcting any mistakes.
Database-related errors are another challenge faced by developers when working with Laravel apps. These can include connection failures, query syntax issues, or integrity constraint violations. Implementing proper error handling mechanisms such as retrying failed queries or rolling back transactions will ensure data consistency and reliability.
Exceptions thrown during runtime require careful attention since they may indicate underlying problems within your codebase or dependencies being utilized by your app. It’s crucial to catch these exceptions appropriately and provide meaningful feedback for debugging purposes.
By familiarizing yourself with these common types of errors in Laravel apps, you’ll be better equipped to handle them effectively and deliver a more robust application overall.
The Importance of Efficient Error Handling in Scalable Apps
Errors are an inevitable part of any software development process. In Laravel apps, errors can occur due to various reasons such as invalid user inputs, database connection issues, or even server failures. However, the way these errors are handled can greatly impact the performance and scalability of your application.
Efficient error handling is crucial in scalable apps because it ensures that potential issues are addressed promptly and effectively. When errors are not efficiently handled, they can lead to downtime, poor user experience, and even security vulnerabilities.
One important aspect of efficient error handling is proper logging. By logging errors and exceptions with meaningful information, developers can easily identify and debug issues. This not only helps in quickly resolving problems but also provides valuable insights for improving the overall stability of the application.
Another key factor in efficient error handling is providing informative error messages to users. Instead of generic error messages like “Oops! Something went wrong,” providing specific details about the issue will help users better understand what went wrong and how they can resolve it.
Implementing a robust exception handling mechanism allows for graceful degradation when unexpected situations arise. It enables developers to catch specific types of exceptions and handle them accordingly instead of letting them propagate throughout the system uncontrollably.
Incorporating automated monitoring tools into your Laravel app can significantly enhance error detection and resolution processes. These tools continuously monitor system metrics and provide real-time alerts whenever a critical error occurs or certain thresholds are breached.
Strategies for Effective Error Handling in Laravel
Strategies for Effective Error Handling in Laravel:
1. Logging and Monitoring: One of the key strategies for effective error handling in Laravel is to implement robust logging and monitoring mechanisms. By logging errors, you can easily track down the source of issues and troubleshoot them efficiently. Additionally, setting up a monitoring system allows you to proactively detect errors before they escalate into major problems.
2. Custom Error Pages: When an error occurs, it’s essential to provide users with a helpful and user-friendly error page instead of displaying default server errors. By customizing error pages, you can offer relevant information about the issue and guide users on what steps to take next.
3. Exception Handling: Exception handling plays a crucial role in ensuring efficient error management in Laravel apps. Utilize try-catch blocks to catch exceptions and handle them appropriately based on their type or severity level.
4. Graceful Degradation: In scalable applications, it’s important to design your codebase in such a way that it gracefully degrades when an unforeseen error occurs rather than crashing completely. This means implementing fallback mechanisms or alternative routes so that users can still access essential functionality even if certain features are temporarily unavailable.
5. Error Reporting and Notifications: Implementing automated systems for reporting errors enables developers to quickly identify issues as they arise, allowing for prompt resolution before they impact users’ experience negatively.
By following these strategies, you can ensure efficient handling of errors within your Laravel applications while maintaining optimal performance and user satisfaction.
Tips for Preventing Errors in Your Laravel App
1. Validate User Input:
One of the most common causes of errors in Laravel apps is invalid user input. To prevent this, always validate user input before processing it. Use Laravel’s built-in validation rules or create custom ones to ensure that only valid data is accepted.
2. Implement Error Logging:
Logging errors can greatly help in identifying and fixing issues in your app. Configure Laravel to log errors and exceptions to a file or a database so that you can easily track them down and take necessary actions.
3. Use Proper Exception Handling:
When an error occurs, it is important to handle it properly instead of letting it crash your entire application. Use try-catch blocks to catch exceptions and gracefully handle them by displaying meaningful error messages or redirecting users to appropriate pages.
4. Test Thoroughly:
Thorough testing is crucial for preventing errors in any application, including Laravel apps. Write unit tests for each component of your app, covering all possible scenarios and edge cases. This will help you identify bugs early on and ensure the stability of your app.
5. Keep Dependencies Updated:
Laravel relies on various third-party libraries and packages, which are regularly updated with bug fixes and security patches. Make sure you keep these dependencies up-to-date to avoid potential conflicts or vulnerabilities that could lead to errors.
6 . Optimize Database Queries:
Inefficient database queries can slow down your app’s performance or even cause unexpected errors due to resource limitations. Optimize your database queries by using indexes where needed, avoiding unnecessary joins or subqueries, caching frequently used data, etc.
Best Practices for Continuous Monitoring and Maintenance
Continuous monitoring and maintenance are crucial aspects of ensuring the smooth functioning and optimal performance of your Laravel application. By implementing best practices in this area, you can proactively identify and resolve any issues or errors that may arise, thus minimizing downtime and providing a seamless experience for your users.
One important practice is to regularly monitor your application’s logs. Log files provide valuable insights into the inner workings of your application, allowing you to track errors, debug issues, and analyze performance metrics. By reviewing these logs on a regular basis, you can quickly identify any potential problems before they escalate into major issues.
It is essential to implement automated testing procedures. This involves creating test cases that simulate various scenarios within your application and verifying that the expected outcomes are achieved. Automated tests help catch bugs early in the development process, reducing the likelihood of encountering them in production.
Regularly updating dependencies is another key practice for maintaining a healthy Laravel app. Keeping up-to-date with framework updates and package versions ensures that you have access to bug fixes, security patches, and new features. It also helps prevent compatibility issues between different components of your application stack.
Implementing proper error handling mechanisms is vital for continuous monitoring as well. By gracefully handling exceptions that may occur during runtime using Laravel’s exception handling system or custom error handlers if necessary – you can ensure smoother operation even when unexpected situations arise.
Lastly but equally important is having a robust backup strategy in place. Regularly backing up both codebase and database allows you to recover from catastrophic failures or data loss efficiently. Consider implementing automated backups through cloud services or dedicated backup tools for added convenience.
By following these best practices for continuous monitoring and maintenance in Laravel applications consistently, developers can keep their apps running smoothly with minimal disruptions while staying proactive about identifying and resolving potential issues promptly.
Conclusion
Efficient error handling is crucial for ensuring the smooth operation of scalable Laravel apps. By understanding common types of errors and implementing effective strategies, developers can improve the user experience and minimize downtime.
We explored various aspects of error handling in Laravel apps. We discussed the importance of efficient error handling, highlighted common types of errors that occur in such applications, and provided strategies for effective error handling.
We shared some useful tips for preventing errors in your Laravel app and emphasized the significance of continuous monitoring and maintenance to ensure optimal performance.
Remember that a proactive approach to identifying and addressing errors is key to maintaining a reliable application. Regularly reviewing logs, conducting thorough testing, and leveraging tools like exception handlers can greatly enhance your ability to detect issues early on.
By following best practices for error handling in Laravel apps, you’ll be well-equipped to create robust applications that deliver exceptional user experiences. So go ahead, implement these strategies in your next project, and take your Laravel development skills to new heights! Happy coding!