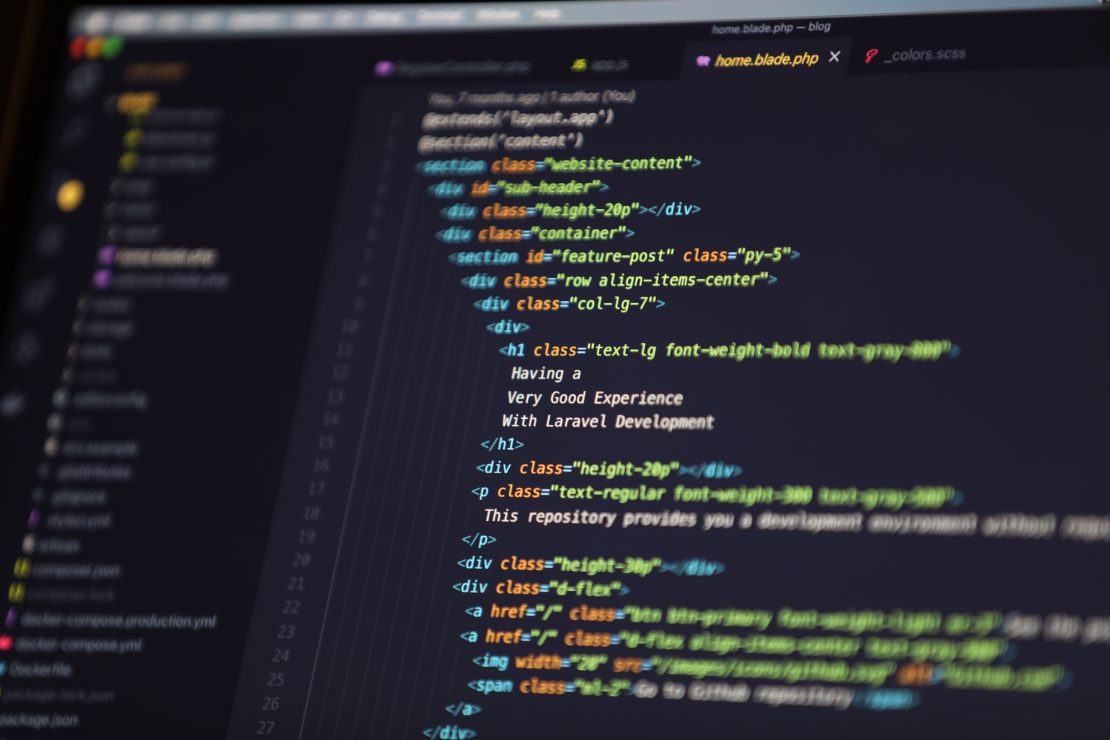
Handling large-scale inventory in an e-commerce system with Laravel requires a robust and scalable approach. Laravel, being a powerful PHP framework, provides the tools needed to manage complex inventory systems efficiently. By following these steps, you can build a scalable and efficient inventory management system using Laravel, ensuring that your e-commerce platform can handle large-scale inventory with ease. Regularly update and optimize your system based on evolving requirements and industry best practices.
The Benefits of using Laravel’s Package Development for E-commerce Extensions
Handling large-scale inventory with Laravel provides several benefits, leveraging the framework’s features and best practices for efficient and scalable inventory management in e-commerce applications. Laravel’s Eloquent ORM simplifies database interactions, making it easier to manage complex relationships and queries associated with large-scale inventory.
Laravel’s built-in queue system allows for asynchronous processing, enabling the system to handle large-scale operations without affecting the user experience. Laravel Horizon provides a dashboard for monitoring and managing queues, enhancing scalability. Laravel’s event broadcasting facilitates real-time updates on inventory changes, enhancing the user experience by providing instant information.
Laravel’s job queues enable efficient batch processing, allowing bulk updates to inventory levels and other resource-intensive tasks. Laravel’s API resource feature simplifies the creation of RESTful APIs, enabling seamless communication between the inventory system and other parts of the application.
Laravel’s secure authentication mechanisms ensure that API endpoints are protected from unauthorized access. Laravel supports various cache drivers, allowing developers to implement caching mechanisms for frequently accessed inventory data, improving performance.
Laravel’s integration with PHPUnit facilitates thorough testing, ensuring the correctness of inventory-related functionalities and preventing potential issues. Laravel supports integration testing, allowing developers to validate the end-to-end flow of inventory management.
Laravel includes built-in Cross-Site Request Forgery (CSRF) protection, enhancing the security of inventory-related operations. Laravel’s validation features ensure that data entering the system meets specified criteria, reducing the risk of data inconsistencies and security vulnerabilities.
Handling large-scale inventory with Laravel not only benefits from the framework’s technical capabilities but also from the supportive community and a well-established ecosystem. These advantages contribute to the development of robust, scalable, and maintainable inventory management systems in the context of e-commerce applications.
Step-by-step guide on How to Implement Laravel’s Package Development for E-commerce Extensions
Handling large-scale inventory with Laravel involves implementing a robust and scalable system to manage products, track stock levels, process orders, and handle various aspects of inventory management efficiently. Here’s a step-by-step guide to help you handle large-scale inventory with Laravel:
1. Database Design:
Design a database schema that includes tables for products, variations, categories, orders, transactions, and any other relevant entities. Define relationships between tables using Laravel’s Eloquent ORM.
2. Product Management:
Create CRUD operations for managing products, including features like product variations, categories, and attributes. Implement forms and validation for adding and updating products.
3. Inventory Tracking:
Implement an inventory tracking system to manage stock levels, variations, and SKU information. Update stock levels on product purchase and track stock movements.
4. Order Management:
Develop order management features to process orders, update inventory levels, and track order fulfillment. Implement order status tracking to manage the entire order lifecycle.
5. Real-time Updates:
Use Laravel’s event broadcasting or a WebSocket library to provide real-time updates on inventory changes to users and administrators. Implement notifications for instant updates on stock levels.
6. Warehouse Management:
If applicable, incorporate warehouse management features to track inventory across multiple locations. Implement logic to fulfill orders from the most optimal warehouse.
7. RESTful API:
Create a RESTful API for seamless communication between the inventory system and other parts of the application. Implement secure authentication for API endpoints.
8. Batch Processing:
Implement batch processing for large-scale operations like updating inventory levels in bulk or processing data imports. Utilize Laravel’s job queues to handle resource-intensive tasks asynchronously.
9. Caching:
Implement caching mechanisms to store frequently accessed inventory data, reducing database queries and improving performance. Utilize Laravel’s caching features for seamless integration.
By following these steps, you can build a comprehensive and scalable inventory management system using Laravel. This approach ensures that your e-commerce platform can efficiently handle large-scale inventory, providing a seamless experience for both administrators and end-users. Regularly update and optimize your system based on evolving requirements and industry best practices.
Best Practices for Handling Large Scale Inventory with Laravel
Handling large-scale inventory with Laravel requires adherence to best practices to ensure efficiency, scalability, and maintainability of the inventory management system. Use appropriate indexes on columns frequently used in queries to improve database performance.
Follow database normalization principles to avoid data redundancy and ensure data integrity. Use Laravel’s eager loading to fetch related data in a single query, reducing the number of database queries. Retrieve only the necessary columns from the database to optimize query performance.
Implement caching mechanisms to store and retrieve frequently accessed data, reducing database queries. Laravel provides cache tags for grouping related items, making it easier to manage and clear cached data. Use Laravel’s queue system to process tasks like updating inventory levels in the background, preventing delays in user interactions.
Prioritize critical jobs to ensure that essential tasks are processed promptly. Implement WebSockets for real-time updates on inventory changes, providing a responsive user experience. Utilize Laravel’s event broadcasting to push real-time updates to connected clients.
If dealing with large-scale operations, break down tasks into smaller batches to prevent resource exhaustion. If dealing with large-scale operations, break down tasks into smaller batches to prevent resource exhaustion. Implement batch processing for data imports and exports to handle large datasets efficiently.
Consider a microservices architecture if dealing with complex inventory processes, allowing for independent scaling of components. Employ load balancing to distribute incoming traffic across multiple servers, ensuring optimal performance. Design API endpoints following RESTful principles for a clear and standardized interface.
Use Laravel’s validation features to validate user input, preventing security vulnerabilities. Implement secure communication between components, especially if the inventory system interacts with external services.
By following these best practices, you can build a high-performance, scalable, and reliable inventory management system using Laravel. Regularly review and update your practices based on evolving requirements and changes in the technology landscape.