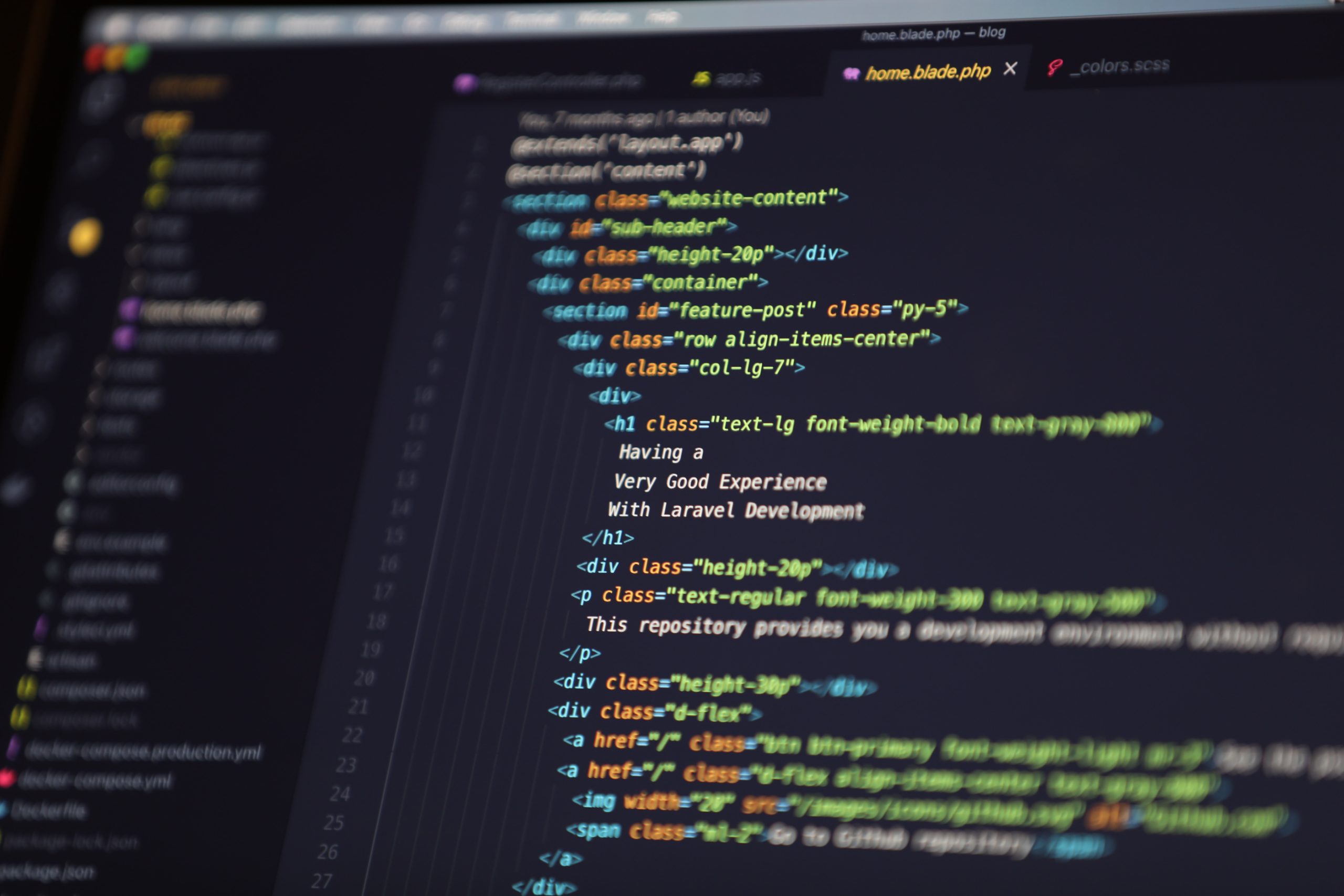
Unleashing the power of Laravel’s high-performance database design is like unlocking a secret weapon for your web applications. In today’s fast-paced digital world, where every millisecond counts, having a well-optimized and efficient database can make all the difference in delivering lightning-fast user experiences.
So, whether you’re building an e-commerce platform handling thousands of transactions per second or creating a content-rich application with millions of records, understanding how to design and optimize your database in Laravel is crucial. In this blog post, we’ll dive into the world of high-performance database design techniques specific to Laravel and explore best practices that will elevate your application’s speed and efficiency.
Get ready to supercharge your app as we delve into the realm of optimizing queries, leveraging caching strategies, scaling databases gracefully, and more! So buckle up – it’s time to take your Laravel applications to new heights!
The Importance of High-Performance Databases
When it comes to building web applications, the importance of high-performance databases cannot be overstated. A well-designed and optimized database can significantly impact the overall speed and efficiency of your application.
A high-performance database ensures that data retrieval and storage operations are executed quickly and seamlessly. This means that users will experience faster load times, resulting in a better user experience. Additionally, with an efficient database design, you can handle large amounts of data without sacrificing performance.
High-performance database is crucial for scalability. As your application grows and handles more users or processes more data, you need a database system that can handle the increased workload without slowing down or crashing.
In addition to improving speed and scalability, optimizing your database also has financial benefits. By reducing the time it takes to process queries or fetch data from the database, you can save on server costs as fewer resources are required to handle the same amount of work.
Investing time in designing and optimizing your database for high performance is essential for any Laravel application. It not only improves user experience but also provides cost savings in terms of server resources needed to run your application efficiently. So don’t overlook this critical aspect when developing your next project!
Best Practices for Database Design
When it comes to database design in Laravel, following best practices is essential for ensuring high performance and efficiency. Here are some key guidelines to consider when designing your database structure:
1. Normalize your data: Normalization helps eliminate redundancy and improve data integrity by breaking down the information into smaller, related tables. This not only reduces storage space but also enables efficient querying and indexing.
2. Optimize table relationships: Define proper relationships between tables using foreign keys and indexes. This allows for faster retrieval of related data and ensures referential integrity.
3. Choose the right data types: Select appropriate data types based on the nature of your data to minimize storage requirements and enhance query performance.
4. Index strategically: Identify frequently queried columns or fields that are used in joins, sorting, or filtering operations, and create indexes accordingly. Be mindful though as excessive indexing can negatively impact write performance.
5. Avoid unnecessary queries: Reduce the number of queries by leveraging eager loading techniques such as lazy loading or eager loading through relationships with Eloquent ORM.
6. Use transactions effectively: Utilize database transactions when performing multiple operations within a single request to ensure atomicity and maintain consistent state despite any errors that may occur during the process.
7. Implement access controls: Apply appropriate access controls at both the application level (using Laravel’s authorization features) and database level (using user roles/permissions) to safeguard sensitive information from unauthorized access.
By adhering to these best practices, you can optimize your database design in Laravel for improved performance, scalability, and reliability without compromising on security or functionality.
Utilizing Eloquent ORM for Efficient Database Operations
When it comes to efficient database operations in Laravel, one tool that stands out is the Eloquent ORM. This powerful feature allows developers to interact with databases using object-oriented syntax, making it easier to write and maintain code.
One of the key benefits of using Eloquent ORM is its ability to abstract away complex SQL queries. Instead of writing raw SQL statements, you can work with models and relationships. This not only improves readability but also reduces the chance of errors.
Another advantage of Eloquent ORM is its support for eager loading. By eagerly loading related data, you can minimize the number of database queries needed to retrieve information. This significantly improves performance by reducing latency.
Eloquent provides a range of methods for filtering and sorting data. Whether you need simple WHERE clauses or more advanced query constraints, this ORM has got you covered. You can easily chain methods together to build complex queries without sacrificing readability.
Apart from querying data efficiently, Eloquent makes it straightforward to perform insertions, updates, and deletions on your database records. With just a few lines of code, you can create new records or update existing ones without having to worry about low-level details like generating SQL statements.
Caching Strategies for Improved Performance
When it comes to optimizing the performance of your Laravel database, implementing effective caching strategies can make a significant difference. Caching involves storing frequently accessed data in memory instead of fetching it from the database every time.
One popular caching strategy is using Redis, an open-source in-memory data structure store. By utilizing Redis as a cache driver in Laravel, you can greatly enhance the speed and efficiency of your application. Redis allows you to store key-value pairs in memory, reducing the need for expensive database queries.
Another valuable caching technique is query result caching. With this approach, instead of executing complex or resource-intensive queries repeatedly, you can store the results temporarily and retrieve them when needed again. This not only reduces database load but also improves response times for users.
Laravel’s built-in cache system provides various methods such as remember(), rememberForever(), and cache tags that enable easy implementation of these caching strategies. Additionally, integrating third-party libraries like Memcached or Varnish can further optimize your application’s performance by caching HTML responses or entire pages.
By leveraging appropriate caching strategies tailored to your specific use cases, you can significantly improve the performance and responsiveness of your Laravel application – resulting in happier users and reduced server load.
Scaling and Optimizing Databases in Laravel
Scaling and optimizing databases in Laravel is crucial for ensuring high performance and efficiency in your web applications. As your application grows and handles larger amounts of data, it becomes necessary to scale your database infrastructure accordingly.
One way to optimize your database is by indexing the appropriate columns. Indexing allows the database engine to quickly locate the desired data, resulting in faster query execution times. It’s important to carefully analyze your application’s queries and determine which columns should be indexed for optimal performance.
Another strategy for scaling and optimizing databases is through horizontal partitioning or sharding. This involves breaking up a large table into smaller, more manageable pieces that can be distributed across multiple servers. By doing so, you can distribute the workload evenly and improve overall query response times.
Implementing proper caching mechanisms can greatly enhance database performance. Leveraging tools like Redis or Memcached can help store frequently accessed data in memory, reducing the need for repeated expensive database queries.
When it comes to scaling databases in Laravel, another approach is using master-slave replication. This involves having one master server that handles write operations while multiple slave servers handle read operations. This setup allows for better distribution of workloads and improved scalability.
Regularly monitoring and optimizing your database’s configuration settings such as buffer sizes, connection limits, and query cache size can have a significant impact on its overall performance.
Conclusion
We have explored the world of high-performance database design in Laravel. We began by understanding the importance of having a well-designed and optimized database for efficient application performance. Then, we delved into best practices for designing databases in Laravel, including proper table structure and indexing.
We also discussed how to leverage the power of Eloquent ORM to simplify database operations and improve overall performance. With its intuitive syntax and powerful features, Eloquent makes it easy to interact with databases while maintaining code readability.
Another aspect we covered was caching strategies for improved performance. By implementing caching mechanisms such as Redis or Memcached, you can significantly reduce database queries and speed up response times.
We touched on scaling and optimizing databases in Laravel. As your application grows, it’s essential to ensure that your database can handle increased traffic without sacrificing performance. From horizontal scaling techniques like sharding to vertical scaling methods like adding more resources to your server, there are various ways to optimize your database’s scalability.
By following these best practices and utilizing the tools provided by Laravel, you can create high-performance databases that ensure your application runs efficiently even under heavy loads.
Remember that every project is unique, so it’s crucial to analyze specific requirements before implementing any optimizations or design decisions. Continuously monitor your application’s performance using profiling tools like Xdebug or Blackfire.io so that you can identify bottlenecks early on and make necessary adjustments.
With a solid understanding of high-performance database design in Laravel along with continuous monitoring and optimization efforts, you can build robust applications that deliver exceptional user experiences.