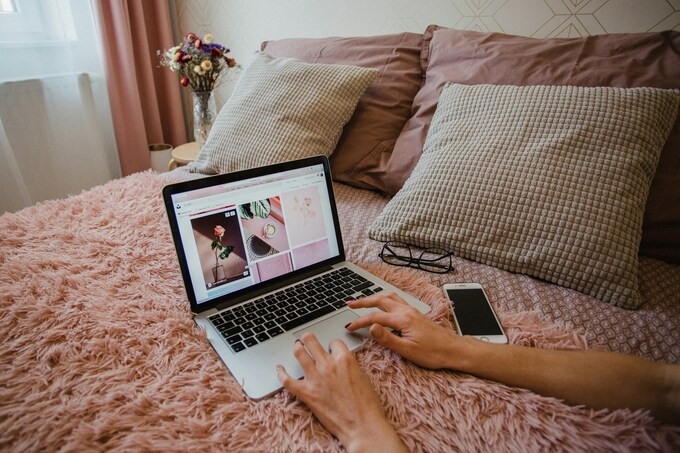
Welcome to the world of web security where protecting your applications from malicious attacks is crucial. One such threat that often lurks in the shadows is Cross-Site Request Forgery (CSRF). In this blog post, we will dive into the realm of CSRF protection specifically tailored for Laravel applications. So, buckle up as we unravel the importance of CSRF protection and walk you through implementing it like a pro in your Laravel projects!
Understanding Cross-Site Request Forgery (CSRF)
Cross-Site Request Forgery, known as CSRF, is a type of malicious attack where unauthorized commands are transmitted from a user that the website trusts. It often occurs when a user is tricked into executing unwanted actions without their knowledge. This can lead to serious security vulnerabilities within web applications.
Hackers exploit CSRF by leveraging the trust that a website has in its users’ browsers. By crafting deceptive requests and causing users to unknowingly execute them while authenticated on a different site, attackers can manipulate sensitive data or perform unauthorized actions.
Understanding how CSRF operates is crucial for developers and website owners to protect their platforms against such attacks. Implementing proper measures like token-based validation helps prevent unauthorized requests from being executed successfully, ensuring the integrity and security of web applications.
The Importance of CSRF Protection in Web Applications
In today’s digital landscape, web applications play a crucial role in our daily lives. However, with the rise of cyber threats, ensuring the security of these applications is paramount. One such threat that developers need to be wary of is Cross-Site Request Forgery (CSRF).
CSRF attacks occur when a malicious actor tricks a user into unknowingly executing actions on a website without their consent. This can lead to unauthorized transactions, data breaches, or even account takeovers.
Implementing CSRF protection in web applications helps prevent these attacks by generating unique tokens for each user session. These tokens verify the authenticity of requests sent to the server, thus safeguarding sensitive operations and data.
By prioritizing CSRF protection in your web application development process, you not only enhance security but also build trust with your users. It showcases your commitment to keeping their information safe and secure.
How Does Laravel Handle CSRF Protection?
Laravel, a popular PHP framework, takes CSRF protection seriously. By default, Laravel generates a CSRF token for each active user session. This token is then added to forms and AJAX requests within the application.
When a form is submitted or an AJAX request is made, Laravel automatically verifies that the CSRF token matches the one stored in the user’s session. If they do not match, Laravel will reject the request as potentially malicious.
To make implementing CSRF protection even easier, Laravel provides middleware that can be applied globally to all routes or selectively to specific routes where it’s needed most.
This built-in functionality helps developers secure their applications against unauthorized actions initiated by malicious third parties without having to manually handle every aspect of CSRF protection themselves.
Steps to Implement CSRF Protection in a Laravel Application
To implement CSRF protection in a Laravel application, the first step is to generate a CSRF token using the `@csrf` Blade directive in your forms. This token will help verify that the authenticated user is indeed the one making the request.
Next, ensure that your routes are protected by middleware by adding `VerifyCsrfToken::class` to the `$middleware` property within your `app/Http/Kernel.php` file. This will automatically validate incoming requests for a valid CSRF token.
Remember to include the CSRF token in all outgoing AJAX requests. You can do this by setting up global AJAX event handlers or including it manually in each request header.
Consider refreshing the CSRF token periodically to prevent potential attacks. You can achieve this by calling `csrf_field()` within your Blade templates or using JavaScript to update it dynamically.
Test your implementation thoroughly to ensure that all forms and AJAX requests are properly protected against CSRF attacks before deploying your Laravel application live.
Best Practices for CSRF Protection in Laravel
When it comes to implementing CSRF protection in Laravel applications, there are some best practices that developers should follow to ensure the security of their web applications.
Always use Laravel’s built-in CSRF protection middleware by including the `csrf` middleware in your application’s HTTP kernel. This will automatically generate and verify tokens for each incoming request, protecting against CSRF attacks.
Make sure to include the `@csrf` Blade directive in your HTML forms. This will insert a hidden CSRF token field that Laravel uses to verify the authenticity of the form submission.
It is also recommended to set up proper CORS (Cross-Origin Resource Sharing) headers in your application to prevent unauthorized cross-origin requests that could bypass CSRF protection.
Regularly update your Laravel framework and dependencies to patch any security vulnerabilities that could potentially expose your application to CSRF attacks. Stay vigilant and proactive in maintaining a secure environment for your users.
Conclusion
Implementing CSRF protection in Laravel applications is crucial for ensuring the security and integrity of your web application. By understanding what CSRF is, why it’s important, and how Laravel handles CSRF protection, you can take the necessary steps to safeguard your application from malicious attacks.
Remember to follow the best practices for implementing CSRF protection in Laravel, such as using Laravel’s built-in features like middleware and tokens, avoiding unnecessary exposure of sensitive actions through GET requests, and regularly updating your dependencies to stay ahead of potential vulnerabilities.
By taking proactive measures to implement CSRF protection in your Laravel application, you can significantly reduce the risk of unauthorized access and data breaches. Stay vigilant, stay informed, and keep prioritizing security in all aspects of your development process.