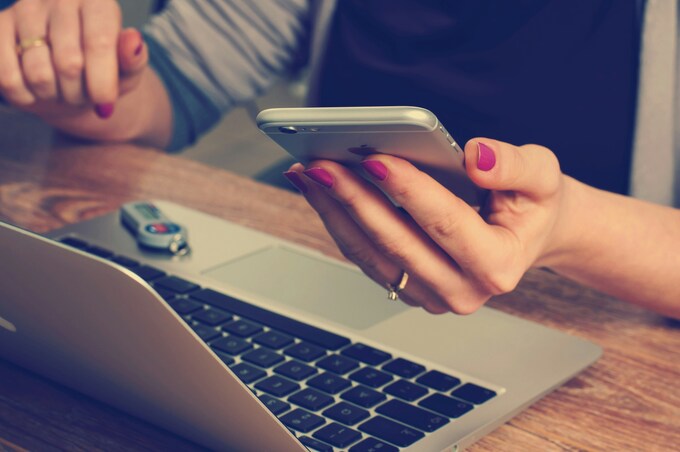
Welcome to the world of Laravel, where time-saving development practices meet elegant code organization! If you’re diving into web development using Laravel framework, understanding the MVC architecture is key to building efficient and scalable applications. In this blog post, we’ll explore how MVC works in Laravel, its benefits, components, implementation steps, optimization tips – everything you need to streamline your coding process. Let’s unravel the magic of MVC in Laravel together!
What is MVC Architecture?
MVC, short for Model-View-Controller, is a software design pattern that separates an application into three interconnected components. The Model represents the data and business logic of the application – it interacts with the database to retrieve and manipulate information. The View component focuses on presenting the data to users in a user-friendly format – this is what users see and interact with on their screens.
The Controller acts as an intermediary between the Model and View – it processes user input, makes decisions based on that input, and updates both the model’s state and view accordingly. By separating concerns, MVC promotes code reusability, maintainability, and scalability in web development projects. It provides a structured approach to organizing your codebase which can lead to more efficient development workflows.
How it Works in Laravel Framework
Laravel framework follows the MVC architecture to organize code efficiently. When a user interacts with a Laravel application, the request is first directed to the controller. The controller then processes the request, interacts with the model to fetch data from the database, and sends it back to the view.
The model represents your data structures and business logic in Laravel. It interacts directly with the database for CRUD operations without any direct connection to views or controllers. This separation of concerns makes your code more organized and easier to maintain.
The view handles how data is presented to users. In Laravel, Blade templating engine allows you to write simple yet powerful PHP code within your views. This helps in creating dynamic content while maintaining readability.
Understanding how MVC works in Laravel can streamline your development process and make your applications more scalable and maintainable over time.
Benefits of Using MVC Architecture in Laravel
When it comes to developing web applications in Laravel, implementing the MVC architecture brings a plethora of benefits. MVC helps in organizing your codebase by separating concerns into distinct components: Models for data manipulation, Views for user interface design, and Controllers for handling user requests. This separation enhances code readability and maintainability.
Using MVC in Laravel allows for easier collaboration among team members as each component has a specific role, making it simpler to work on different parts of the application simultaneously. Moreover, this architectural pattern promotes reusability of code segments across various projects within the framework.
By adhering to MVC principles in Laravel development, you can streamline testing processes since each component can be tested independently. This results in faster debugging and overall improved software quality.
The Three Components of MVC: Model, View, and Controller
In the MVC architecture, there are three key components: Model, View, and Controller. The Model represents the data layer of the application – it interacts with the database and contains business logic. It is responsible for managing the data and ensuring its integrity.
On the other hand, we have the View which is responsible for presenting the data to users in a user-friendly way. It defines how information should be displayed to users and interacts with them through interfaces.
We have the Controller which acts as an intermediary between the Model and View. It receives input from users via Views, processes it using Models, and then returns output back to Views. Controllers play a crucial role in handling user requests and determining which actions need to be taken based on those requests.
By understanding these three components – Model, View, and Controller – developers can effectively organize their codebase in Laravel applications for better scalability and maintainability.
Implementing MVC in Laravel Step by Step
Are you ready to dive into the world of implementing MVC in Laravel step by step? Let’s start with the Model – this is where your data logic resides. Create a model for each table in your database, defining relationships and handling data manipulation.
Next up, we have the View – this is where you design the user interface. Utilize Blade templates to create dynamic and interactive views that display data from your models beautifully.
And lastly, we have the Controller – acting as the middleman between models and views. Controllers handle user requests, process input, interact with models, and return appropriate responses to the views.
By following these steps diligently, you can ensure a well-structured application that separates concerns efficiently. Stay tuned for more insights on optimizing your code with MVC!
Tips for Optimizing Your Code with the MVC Approach
When implementing the MVC architecture in Laravel, optimizing your code is essential for improving performance and maintainability. One tip is to keep your controllers lean by delegating complex logic to the model layer. This helps in keeping your codebase organized and easier to manage.
Another useful tip is to make use of Laravel’s built-in Eloquent ORM for interacting with the database within your models. By utilizing Eloquent relationships and eager loading, you can streamline database queries and enhance efficiency.
Consider using Blade template caching to reduce server load by storing compiled views for faster retrieval. This can significantly improve the response time of your web application.
Don’t forget about route caching which can drastically speed up the routing process by generating a cached file of all your application’s routes. This optimization technique can lead to quicker page loads and better overall user experience when navigating through your Laravel application.
Conclusion
Adopting the MVC architecture in Laravel can significantly enhance your development process by organizing your codebase into manageable components. By separating concerns and promoting reusability, maintainability, and scalability, MVC helps streamline your workflow and improve the overall quality of your application. Embrace this time-saving approach to elevate your Laravel projects to new heights of efficiency and effectiveness. Cheers to building better software with MVC in Laravel!